Subscribe to Our Newsletter
Sign up for our bi-weekly newsletter to learn about computer vision trends and insights.
Create Your Own Contraband Detector
April 14, 2020 by
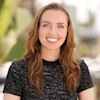
Lila Mullany
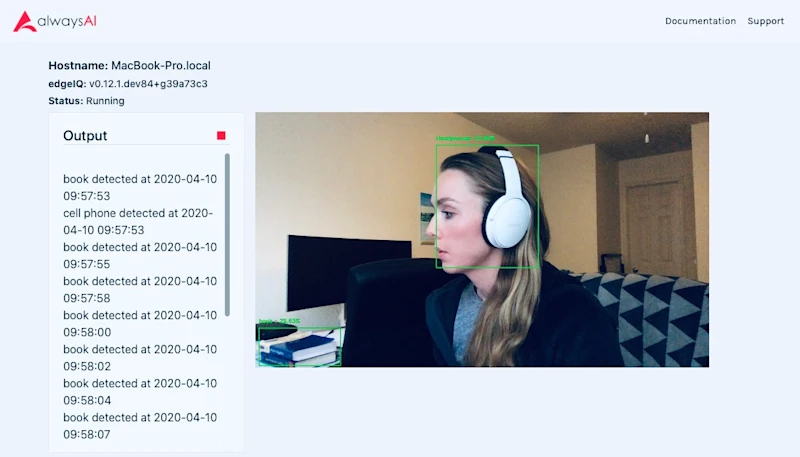
Subscribe to Our Newsletter
Sign up for our bi-weekly newsletter to learn about computer vision trends and insights.
Lila Mullany