Subscribe to Our Newsletter
Sign up for our bi-weekly newsletter to learn about computer vision trends and insights.
How to Build a Simple Computer Vision Texting App Using Twilio
March 19, 2020 by
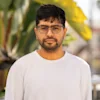
Carlos Lazo
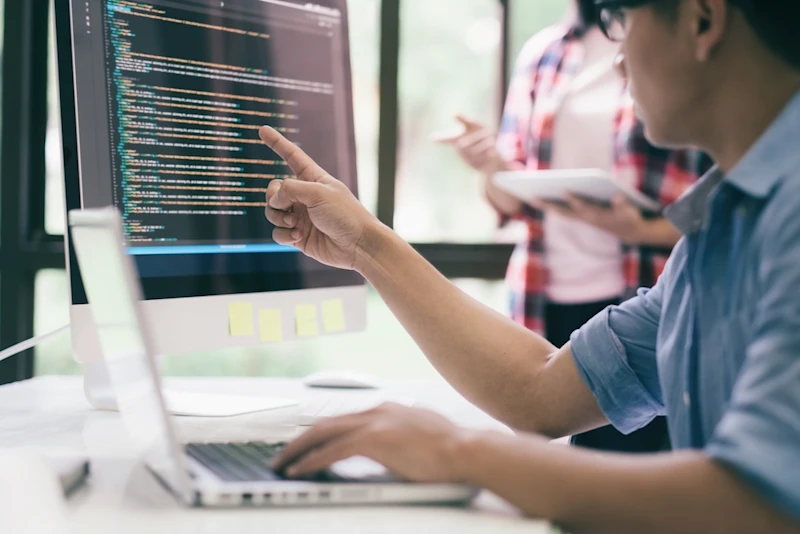
Subscribe to Our Newsletter
Sign up for our bi-weekly newsletter to learn about computer vision trends and insights.
Carlos Lazo